DB를 이용하여 채널 개별 수정하기
유효성 검사
채널 개별 수정의 경우에는 param을 통해 채널번호를 받고, body값으로는 title과 description을 받지만 description은 필수항목이 아니기 때문에 유효성검사에서는 제외하였습니다.
param("id").notEmpty().withMessage("채널번호가 없습니다."),
body("title").notEmpty().withMessage("제목을 입력하세요"),
채널 개별 수정
let { id } = req.params;
id = parseInt(id);
const { title, description } = req.body;
const update_sql ="update channels set title = ? , description = ? where id = ?";
const values = [title, description, id];
try {
const [data] = await conn.query(update_sql, values);
if (data.affectedRows == 0) {
return notFoundChannel(res);
}
res.status(200).json({
message: `채널명이 ${title}(으)로, 수정이 완료되었습니다.`
});
} catch (err)
return res.status(400).end();
}
이전 코드 정리
DB 구성
db = {
idx,
{
user : test1,
channels :[
{channelTitle : '테스트', description : '채널설명', channelId : 번호}
]
}
}
기본 코드 작성
app
.route("/channels/:user/:num")
.put((req, res) => {
let { user, num } = req.params;
const { channelTitle, description } = req.body;
num = parseInt(num);
let hasUser = false;
let channelData = "";
let userKey = "";
db.forEach((v, i) => {
if (user === v.user) {
hasUser = true;
userKey = i;
channelData = v.channels.findIndex((f) => {
if (f.channelId == num) {
return true; // 0 || 양수
} else {
return false; // -1
}
});
}
});
res.status(200).json({
message: "성공적으로 수정되었습니다."
});
})
개별 수정이다 보니 URI로 user와 채널번호를 받아오고, 수정값들은 body를 통해서 받아오게 만들어보았습니다. 이번에는 channelData로 배열의 index 값을 받아오게 만들었는데요. 이때 일치하는 데이터가 있으면 0 이상의 값이 나오고, 일치하는 데이터가 없으면 -1로 음수가 나오는 걸 확인할 수 있었습니다.
Check 사항
1. 유저가 존재하는가?
2. 채널이 존재하는가?
3. 필수 데이터가 존재하는가?(channelTitle)
1. 유저가 존재하는가?
if (!hasUser) {
res.status(404).json({
message: "존재하지 않는 유저입니다."
});
return;
}
hasUser로 false 값일때 404 상태코드와 메시지를 전달하였습니다.
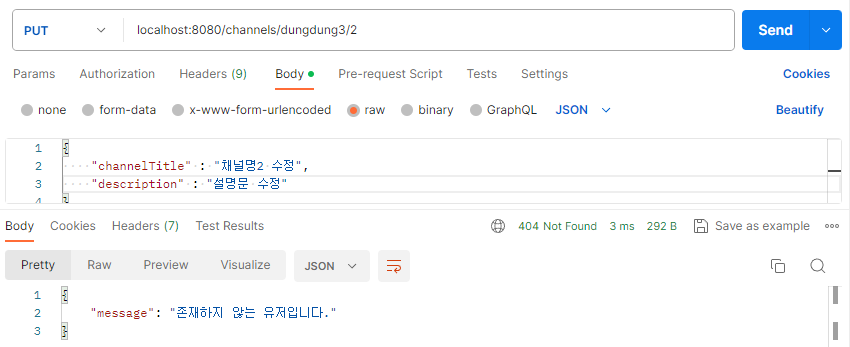
2. 채널이 존재하는가?
if (channelData === -1) {
res.status(404).json({
message: "존재하지 않는 채널입니다."
});
return;
}
channelData가 -1일때, 채널이 존재하지 않는 것이기 때문에 404 상태코드와 메시지를 전달하였습니다.
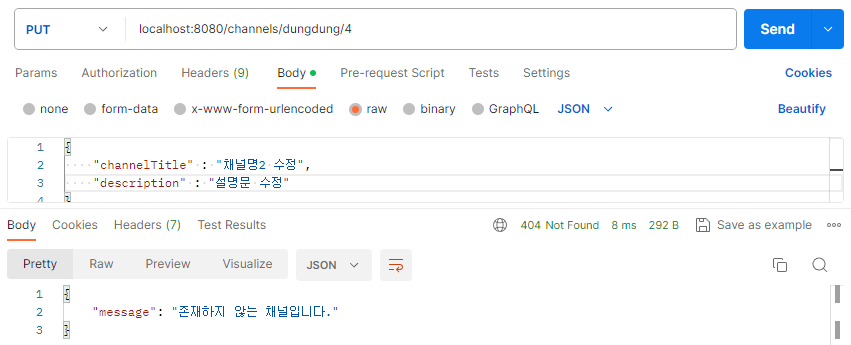
3. 필수 데이터가 존재하는가?(channelTitle)
if (!channelTitle) {
res.status(400).json({
message: "채널명이 비어있습니다."
});
return;
}
채널 개별 수정시에 필수 데이터로 channelTitle 값이 존재해야 하기 때문에 요청에러인 400 코드와 메시지를 전달하였습니다.
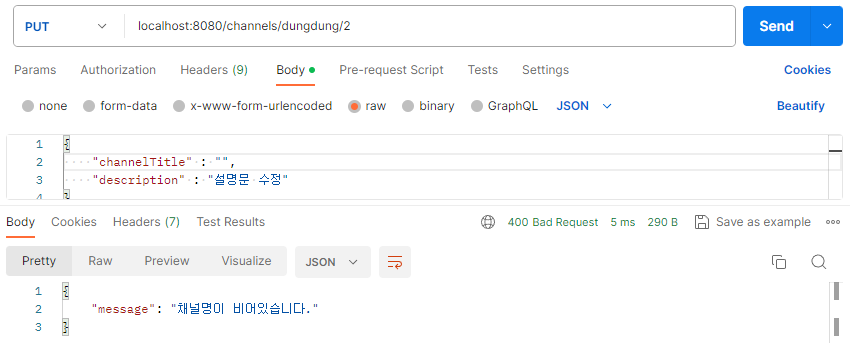
이제 check 사항에 문제가 없다면 데이터를 수정을 해주어야 하는데요.
if (channelTitle) {
db.get(userKey).channels[channelData].channelTitle = channelTitle;
}
db.get(userKey).channels[channelData].description = description;
db의 객체 안의 배열에 접근하여 value값만 변경하였습니다. 이때도 chnnelTitle은 존재할 때 해당 데이터가 들어가게 만들었지만 description은 존재하지 않아도 그대로 값을 덮어쓸 수 있게 만들었습니다.
'프로젝트 > 1.youtube-project' 카테고리의 다른 글
[실전]미니 프로젝트 - 서버에서 router관리하기 (0) | 2023.12.17 |
---|---|
[실전]미니 프로젝트 - 채널 전체 "조회" (0) | 2023.12.16 |
[실전]미니 프로젝트 - 개별 채널 "삭제" (0) | 2023.12.15 |
[실전]미니 프로젝트 - 채널 개별 "조회" (0) | 2023.12.14 |
[실전]미니 프로젝트 - 채널 "생성" (0) | 2023.12.14 |
DB를 이용하여 채널 개별 수정하기
유효성 검사
채널 개별 수정의 경우에는 param을 통해 채널번호를 받고, body값으로는 title과 description을 받지만 description은 필수항목이 아니기 때문에 유효성검사에서는 제외하였습니다.
param("id").notEmpty().withMessage("채널번호가 없습니다."),
body("title").notEmpty().withMessage("제목을 입력하세요"),
채널 개별 수정
let { id } = req.params;
id = parseInt(id);
const { title, description } = req.body;
const update_sql ="update channels set title = ? , description = ? where id = ?";
const values = [title, description, id];
try {
const [data] = await conn.query(update_sql, values);
if (data.affectedRows == 0) {
return notFoundChannel(res);
}
res.status(200).json({
message: `채널명이 ${title}(으)로, 수정이 완료되었습니다.`
});
} catch (err)
return res.status(400).end();
}
이전 코드 정리
DB 구성
db = {
idx,
{
user : test1,
channels :[
{channelTitle : '테스트', description : '채널설명', channelId : 번호}
]
}
}
기본 코드 작성
app
.route("/channels/:user/:num")
.put((req, res) => {
let { user, num } = req.params;
const { channelTitle, description } = req.body;
num = parseInt(num);
let hasUser = false;
let channelData = "";
let userKey = "";
db.forEach((v, i) => {
if (user === v.user) {
hasUser = true;
userKey = i;
channelData = v.channels.findIndex((f) => {
if (f.channelId == num) {
return true; // 0 || 양수
} else {
return false; // -1
}
});
}
});
res.status(200).json({
message: "성공적으로 수정되었습니다."
});
})
개별 수정이다 보니 URI로 user와 채널번호를 받아오고, 수정값들은 body를 통해서 받아오게 만들어보았습니다. 이번에는 channelData로 배열의 index 값을 받아오게 만들었는데요. 이때 일치하는 데이터가 있으면 0 이상의 값이 나오고, 일치하는 데이터가 없으면 -1로 음수가 나오는 걸 확인할 수 있었습니다.
Check 사항
1. 유저가 존재하는가?
2. 채널이 존재하는가?
3. 필수 데이터가 존재하는가?(channelTitle)
1. 유저가 존재하는가?
if (!hasUser) {
res.status(404).json({
message: "존재하지 않는 유저입니다."
});
return;
}
hasUser로 false 값일때 404 상태코드와 메시지를 전달하였습니다.
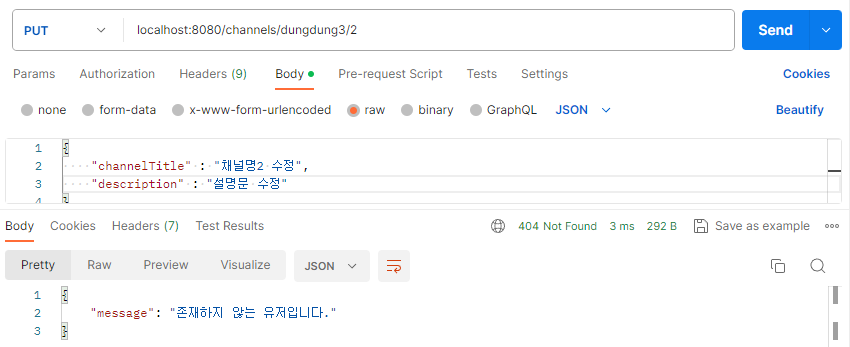
2. 채널이 존재하는가?
if (channelData === -1) {
res.status(404).json({
message: "존재하지 않는 채널입니다."
});
return;
}
channelData가 -1일때, 채널이 존재하지 않는 것이기 때문에 404 상태코드와 메시지를 전달하였습니다.
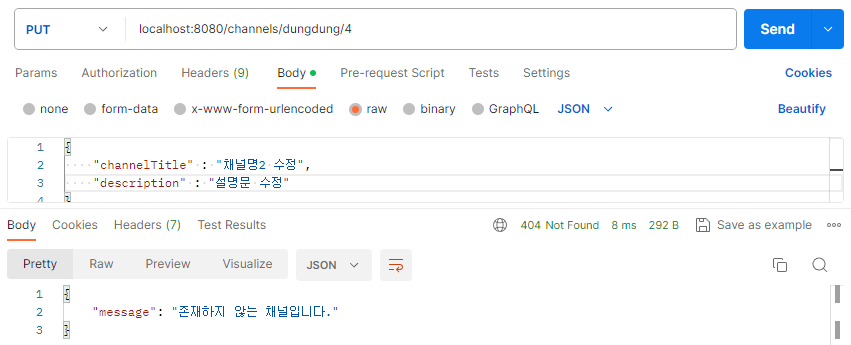
3. 필수 데이터가 존재하는가?(channelTitle)
if (!channelTitle) {
res.status(400).json({
message: "채널명이 비어있습니다."
});
return;
}
채널 개별 수정시에 필수 데이터로 channelTitle 값이 존재해야 하기 때문에 요청에러인 400 코드와 메시지를 전달하였습니다.
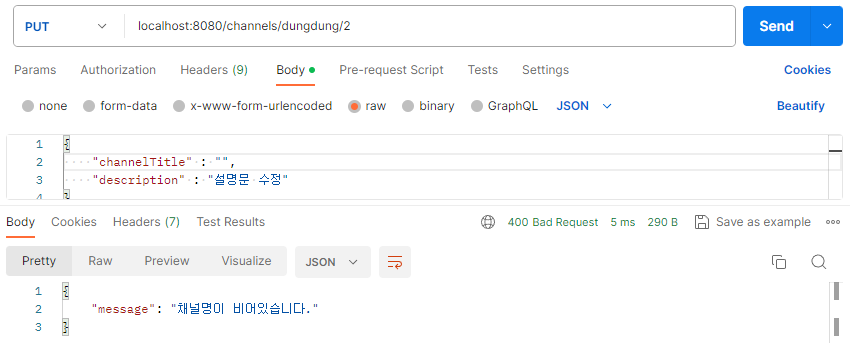
이제 check 사항에 문제가 없다면 데이터를 수정을 해주어야 하는데요.
if (channelTitle) {
db.get(userKey).channels[channelData].channelTitle = channelTitle;
}
db.get(userKey).channels[channelData].description = description;
db의 객체 안의 배열에 접근하여 value값만 변경하였습니다. 이때도 chnnelTitle은 존재할 때 해당 데이터가 들어가게 만들었지만 description은 존재하지 않아도 그대로 값을 덮어쓸 수 있게 만들었습니다.
'프로젝트 > 1.youtube-project' 카테고리의 다른 글
[실전]미니 프로젝트 - 서버에서 router관리하기 (0) | 2023.12.17 |
---|---|
[실전]미니 프로젝트 - 채널 전체 "조회" (0) | 2023.12.16 |
[실전]미니 프로젝트 - 개별 채널 "삭제" (0) | 2023.12.15 |
[실전]미니 프로젝트 - 채널 개별 "조회" (0) | 2023.12.14 |
[실전]미니 프로젝트 - 채널 "생성" (0) | 2023.12.14 |